iOS - 文本字段
文本字段的使用
文本字段是使应用能够获取用户输入的 UI 元素。
一个 UITextfield 如下所示。
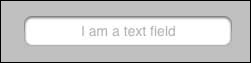
文本字段的重要属性
- 没有用户输入时显示的占位符文本
- 普通文本
- 自动修正类型
- 键盘类型
- 回车键类型
- 清空按键模式
- 对齐方式
- 委托
在 xib 中更新属性
您可以在实用工具区域(窗口右侧)的属性检查器中更改 xib 中的文本字段属性。
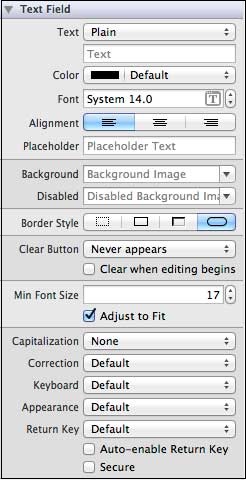
文本字段委托
我们可以在界面生成器中通过右键单击 UIElement 来设置委托,并将其连接到文件所有者,如下所示。
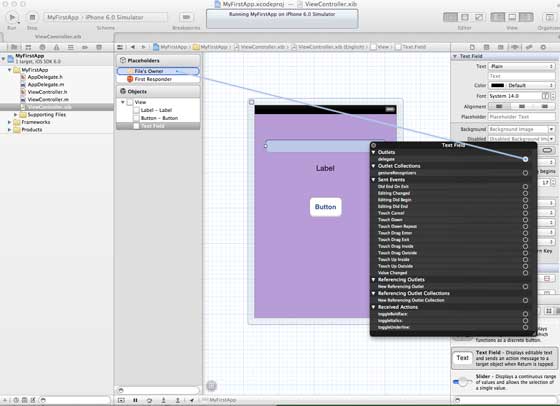
使用委托的步骤
步骤 1 − 设置委托如上图。
步骤 2 − 添加您的类响应的委托。
步骤 3 − 实现 textField Delegates,重要的文本字段委托如下 −
- (void)textFieldDidBeginEditing:(UITextField *)textField - (void)textFieldDidEndEditing:(UITextField *)textField
步骤 4 − 顾名思义,一旦我们分别开始编辑文本字段和结束编辑,就会调用上述两个委托。
步骤 5 − 对于其他委托,请参阅 UITextDelegate 协议参考。
示例代码和步骤
步骤 1 − 我们将使用为 UI 元素创建的示例应用程序。
步骤 2 − 我们的 ViewController 类将采用 UITextFieldDelegate 并且我们的 ViewController.h 文件更新如下 −
实例
#import <UIKit/UIKit.h>
// You can notice the adddition of UITextFieldDelegate below
@interface ViewController : UIViewController<UITextFieldDelegate>
@end
步骤 3 − 然后我们将方法 addTextField 添加到我们的 ViewController.m 文件中。
步骤 4 − 然后我们在 viewDidLoad 方法中调用这个方法。
步骤 5 − 更新 ViewController.m 中的 viewDidLoad 如下 −
实例
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
//The custom method to create our textfield is called
[self addTextField];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
-(void)addTextField {
// This allocates a label
UILabel *prefixLabel = [[UILabel alloc]initWithFrame:CGRectZero];
//This sets the label text
prefixLabel.text =@"## ";
// This sets the font for the label
[prefixLabel setFont:[UIFont boldSystemFontOfSize:14]];
// This fits the frame to size of the text
[prefixLabel sizeToFit];
// This allocates the textfield and sets its frame
UITextField *textField = [[UITextField alloc] initWithFrame:
CGRectMake(20, 50, 280, 30)];
// This sets the border style of the text field
textField.borderStyle = UITextBorderStyleRoundedRect;
textField.contentVerticalAlignment = UIControlContentVerticalAlignmentCenter;
[textField setFont:[UIFont boldSystemFontOfSize:12]];
//Placeholder text is displayed when no text is typed
textField.placeholder = @"Simple Text field";
//Prefix label is set as left view and the text starts after that
textField.leftView = prefixLabel;
//It set when the left prefixLabel to be displayed
textField.leftViewMode = UITextFieldViewModeAlways;
// Adds the textField to the view.
[self.view addSubview:textField];
// sets the delegate to the current class
textField.delegate = self;
}
// pragma mark is used for easy access of code in Xcode
#pragma mark - TextField Delegates
// This method is called once we click inside the textField
-(void)textFieldDidBeginEditing:(UITextField *)textField {
NSLog(@"Text field did begin editing");
}
// This method is called once we complete editing
-(void)textFieldDidEndEditing:(UITextField *)textField {
NSLog(@"Text field ended editing");
}
// This method enables or disables the processing of return key
-(BOOL) textFieldShouldReturn:(UITextField *)textField {
[textField resignFirstResponder];
return YES;
}
- (void)viewDidUnload {
label = nil;
[super viewDidUnload];
}
@end
步骤 6 − 当我们运行应用程序时,我们将得到以下输出。
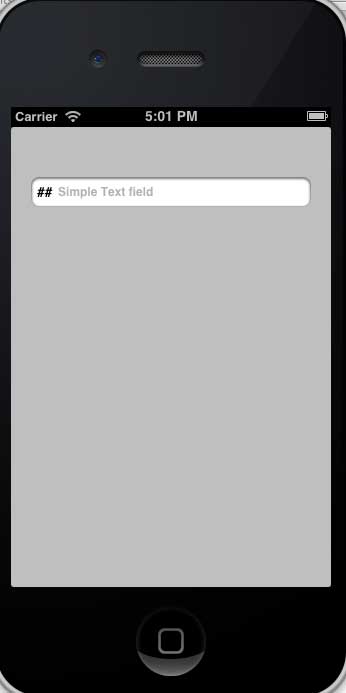
步骤 7 − 委托方法是根据用户操作调用的。 查看控制台输出以了解何时调用代表。